Overview
This project deals with a line follower robot that follows the human-made path. It follows the line and take turns and get stops at the position where the line ends
In this project, we have used photoresistors to sense the path motors to make the robot move an external 9v power source. When the sensor senses the path accordingly the motors rotate this is very useful for us as when we want to move a person on the same path continuously we can use this robot.
Hardware required
- Arduino Uno R3
- Hobby Gear Motor
- Resistor
- Photoresistor
- Jumper Wires
- 9V Battery
- L293D
Schematic Diagram
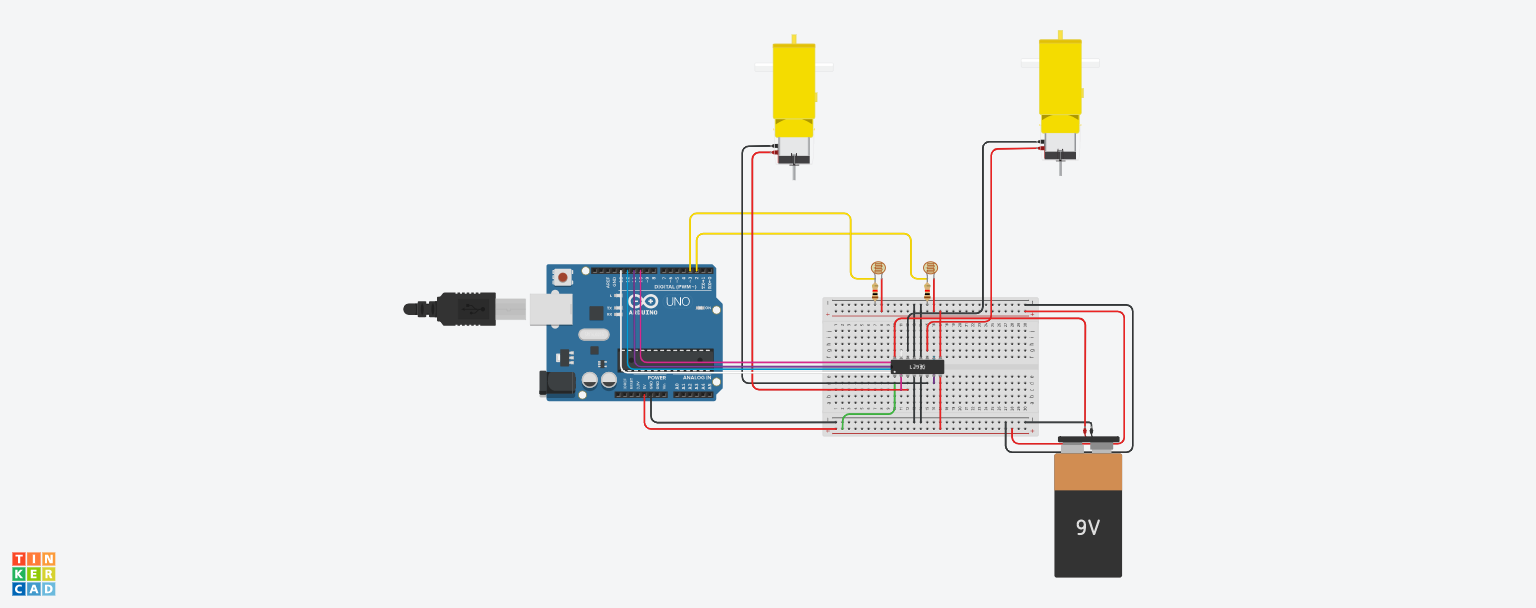
Fig 1. Circuit Diagram
Arduino Code :
#define LS 2 // left sensor
#define RS 3 // right sensor
#define LM1 10 // left motor
#define LM2 11 // left motor
#define RM1 12 // right motor
#define RM2 13 // right motor
void setup()
{
pinMode(LS, INPUT);
pinMode(RS, INPUT);
pinMode(LM1, OUTPUT);
pinMode(LM2, OUTPUT);
pinMode(RM1, OUTPUT);
pinMode(RM2, OUTPUT);
}
void loop()
{
if(digitalRead(LS) && digitalRead(RS)) // Move Forward
{
digitalWrite(LM1, HIGH);
digitalWrite(LM2, LOW);
digitalWrite(RM1, HIGH);
digitalWrite(RM2, LOW);
}
if(!(digitalRead(LS)) && digitalRead(RS)) // Turn right
{
digitalWrite(LM1, LOW);
digitalWrite(LM2, LOW);
digitalWrite(RM1, HIGH);
digitalWrite(RM2, LOW);
}
if(digitalRead(LS) && !(digitalRead(RS))) // turn left
{
digitalWrite(LM1, HIGH);
digitalWrite(LM2, LOW);
digitalWrite(RM1, LOW);
digitalWrite(RM2, LOW);
}
if(!(digitalRead(LS)) && !(digitalRead(RS))) // stop
{
digitalWrite(LM1, LOW);
digitalWrite(LM2, LOW);
digitalWrite(RM1, LOW);
digitalWrite(RM2, LOW);
}
}
Precautions
- Connections should be done properly.
- Arduino is case Sensitive so code accordingly.
- Give different colours to the wires.
- Colour coding to be followed properly.